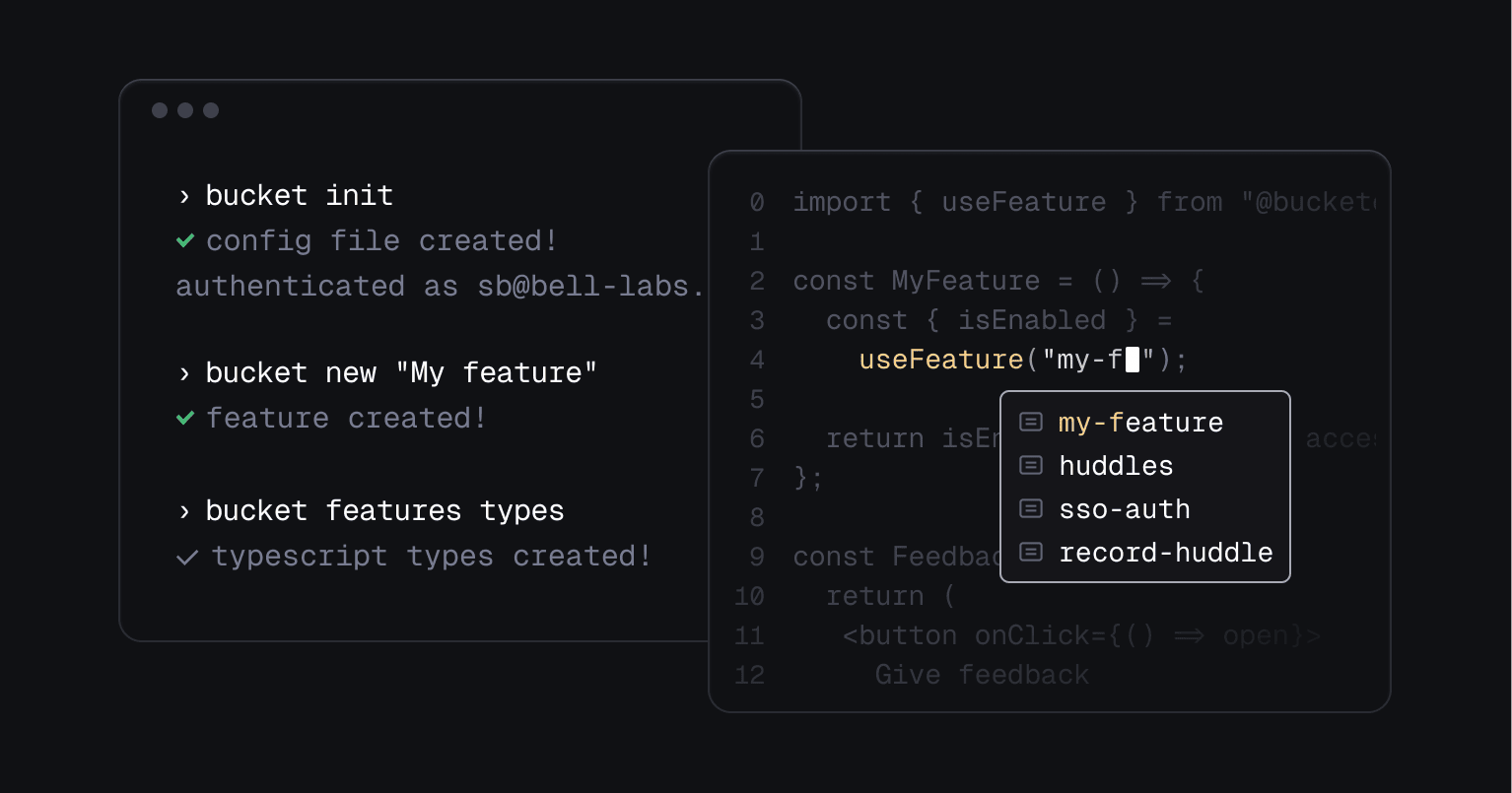
CLI & Type Safety
We have shipped a command line interface (CLI) that you can use to interact with Bucket. The CLI removes any friction in creating features from the command line and helps you maintain type safety for your features:
› npm i @bucketco/cli --save-dev
› bucket new "My Feature"
When creating a new feature, the CLI will automatically update your local types to make sure they match those defined in Bucket, saving you from making frustrating typos referencing your feature keys.
The CLI will also generate types for Remote Config based on the payloads configured in Bucket. This helps ensure that the shape of the payload you’ve configured in Bucket matches what you’re working with in the code.
function MyFeature() {
// The feature key argument passed to the useFeature hook is strongly
// typed and will give a compile-time error if the key does not exist.
const { isEnabled, config } = useFeature("my-feature");
if (!isEnabled) return null;
// Given the following payload configured in Bucket:
// {
// fontWeight: "bold",
// copy: "Sync now!"
// }
//
// The CLI will generate the type:
// {
// fontWeight: string;
// copy: string;
// }
//
// Which will give a compile-time error when we refer to
// the missing "fontBold" property below.
return (
<button style={{fontWeight: config.payload.fontBold}}>
{config.payload.copy}
</button>
)
}
Happy shipping!